Intermediate
An introduction to Unity
This tutorial provides an introduction to Unity, a popular game development platform, and how to integrate it with Ethereum-based technologies such as ERC721 tokens and EVM practices. The tutorial assumes a basic understanding of Unity, ERC721, and EVM practices. The documentation supports WebGL, IOS, and Android builds and recommends using a Mobile Development IDE with Device Simulation Capabilities such as Xcode to test mobile builds. DefiConnect is required for the Login Example, and some scenes require assets to work properly. The tutorial provides instructions on downloading and installing Unity, importing the web3.unity folders into the project, and using EVM methods such as Player Account, Block Number, Balance Of, Verify, Nonce, and Convert WEI to CRO and CRO to WEI. The tutorial also includes information on the Chain Overview and how to import the ChainSafe Package from Github or Releases.
Pre-requisites
This SDK assumes that you have a basic understanding of Unity, ERC721 and EVM practices.
Supported OS
This documentation currently supports WebGL, IOS and Android builds. Other platforms may work but there is no guarantee. We will extend our support to other platforms after we have stabilized our current architecture.
Other Requirements
-
Mobile IDE: If you want to test your Mobile Builds we recommend you to download a Mobile Development IDE with Device Simulation Capabilities such as Xcode.
-
DefiConnect: DefiConnect is required for the Login Example.
-
Assets: Some of the scenes require assets to work properly. If you do not have any assets you can always use the Cronos Testnet and generate some ERC721 test assets. You can read more around integration methods here.
Download and install Unity
You can refer to the Unity Download Section
Unity Build Modules
Depending on your preferred build settings we recommend to install platform packages for WebGL
, IOS
and Android
from your Unity build settings.
Chain Overview
You can use the following RPC methods. By default, we use the mainnet rpc method.
Testnet
-
RPC Method:
https://evm-dev-t3.cronos.org
-
Chain ID: 338
Import ChainSafe Package
Github
You can clone the following repository.
$ git clone git@github.com:ChainSafe/web3.unity.git
Releases
Or you can download the latest releases
Create a new Unity Project
Create a new project directly from your Unity Hub. In the next step Choose 3D as your project type.
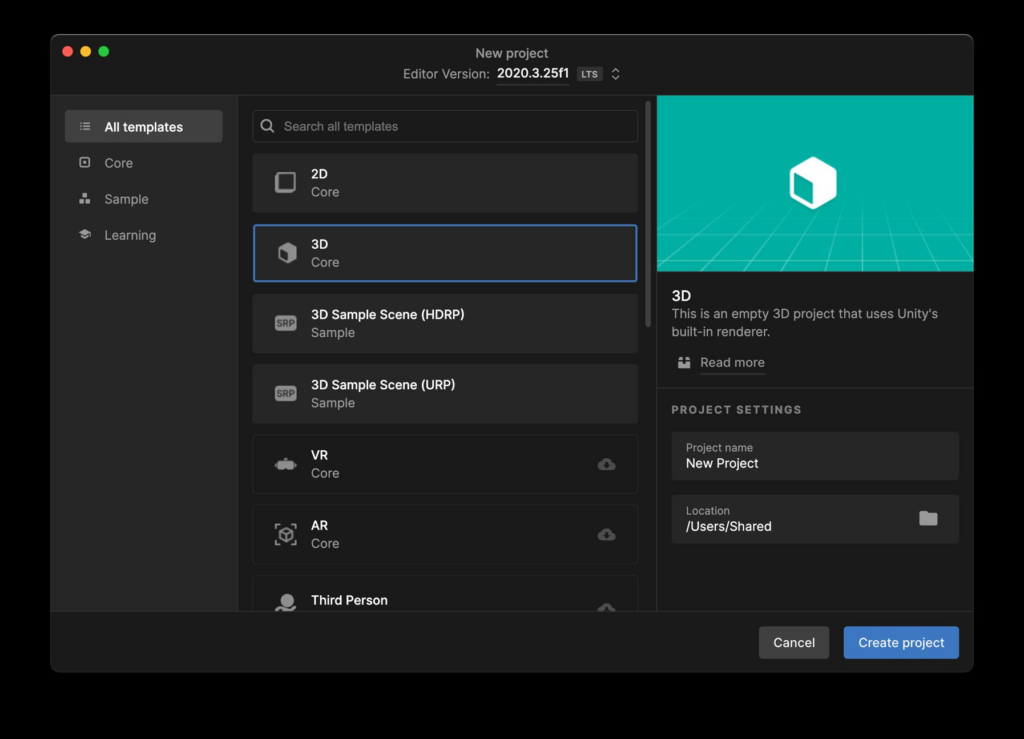
Upload web3.unity Folders into Project
Now you have to import the content of the cloned repository (web3.unity) directly into your newly created project assets folder.
EVM
A standard interface for EVM methods
This section contains the different types of EVM methods that can be used in the Unity SDK. For additional operations and methods please refer to the ChainSafe Documentation.
Player Account
PlayerPrefs.GetString(“Account”) is the user’s wallet account accessed after the LoginScene.
string account = PlayerPrefs.GetString("Account"); print(account);
Important: If you run your script from the editor, you will have to hardcode the account string (e.g. “0x6e…”)
Block number
Get the current latest block number
string chain = "cronos"; string network = "mainnet"; // mainnet or testnet int blockNumber = await EVM.BlockNumber(chain, network); print(blockNumber); Some code
Balance Of
Get the balance of the native blockchain
string chain = "cronos"; string network = "mainnet"; // mainnet or testnet string account = "WALLET_ADDRESS"; string balance = await EVM.BalanceOf(chain, network, account, rpc); print(balance);
Verify
Verify a signed message.
string message = "YOUR_MESSAGE"; string signature = "YOUR_SIGNATURE"; string address = await EVM.Verify(message, signature); print(address);
Nonce
Print Nonce.
string chain = "cronos"; string network = "mainnet"; // mainnet or testnet string account = "WALLET_ADDRESS"; string rpc = "https://evm-dev.cronos.org"; string nonce = await EVM.Nonce(chain, network, account, rpc); print(nonce); chain = "cronos";
Convert WEI to CRO and CRO to WEI
Converts WEI values
float cro = float.Parse("0.1"); float decimals = 1000000000000000000; // 18 decimals float wei = cro * decimals; print(Convert.ToDecimal(wei).ToString()); float wei = float.Parse("10123755"); float decimals = 1000000000000000000; // 18 decimals float cro = wei / decimals; print(Convert.ToDecimal(cro).ToString());