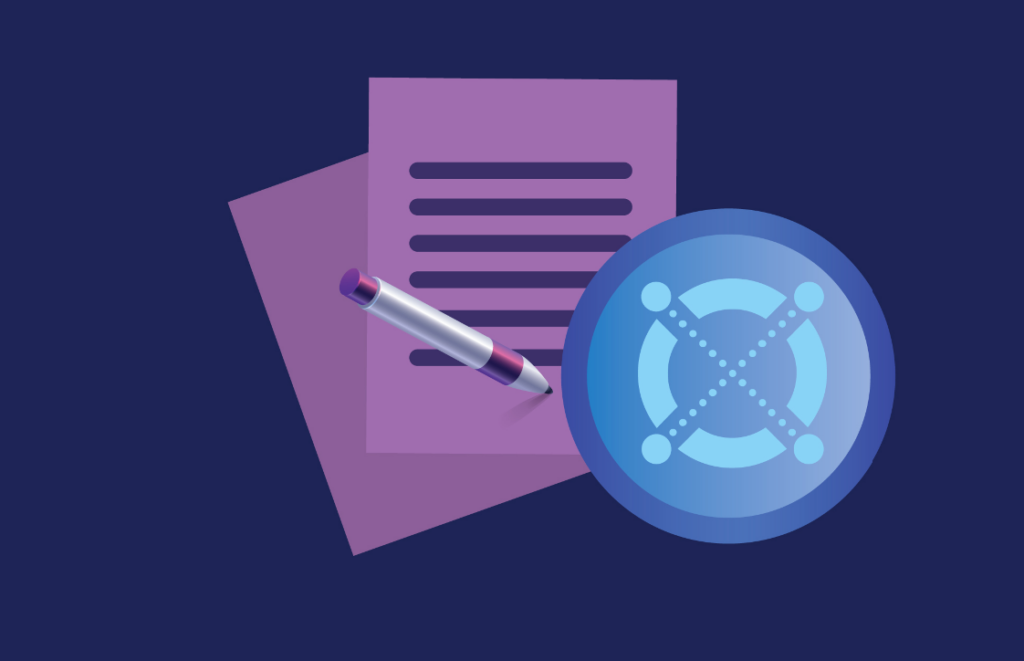
Intermediate
How to create Smart Contract and deploy on MultiversX network
Welcome to this tutorial on how to create and deploy a smart contract on the Elrond network. Smart contracts are self-executing contracts with the terms of the agreement between buyer and seller being directly written into lines of code. They allow for the automation of digital interactions and can be used to facilitate, verify, and enforce the negotiation or performance of a contract. In this tutorial, you will learn how to create and deploy a smart contract on the Elrond network using the Ergo programming language and the Elrond development tools. We will walk you through the steps needed to set up your Elrond development environment, write and compile your smart contract code, and deploy it to the Elrond testnet. Let’s get started!
Prerequisites
You need to have erdpy installed.
Create the contract
In a folder of your choice, run the following command:
erdpy contract new --template="simple-counter" mycounter
This creates a new folder named mycounter
which contains the C source code for a simple Smart Contract – based on the template simple-counter. The file counter.c
is the implementation of the Smart Contract, which defines the following functions:
-
init()
: this function is executed when the contract is deployed on the Blockchain -
increment()
anddecrement()
: these functions modify the internal state of the Smart Contract -
get()
: this is a pure function (does not modify the state) which we’ll use to query the value of the counter
Build the contract
In order to build the contract to WASM, run the following command:
erdpy --verbose contract build mycounter
Above, mycounter
refers to the previously created folder, the one that holds the source code. After executing the command, you can inspect the generated files in mycounter/output
.
Deploy the contract on the Testnet
In order to deploy the contract on the Testnet you need to have an account with sufficient balance (required for the deployment fee) and the associated private key in PEM format.
The deployment command is as follows:
erdpy --verbose contract deploy --project=mycounter --pem="alice.pem" --gas-limit=5000000 --proxy="https://testnet-gateway.elrond.com" --outfile="counter.json" --recall-nonce --send
Above, mycounter
refers to the same folder that contains the source code and the build artifacts. The deploy
command knows to search for the WASM bytecode within this folder.
Note the last parameter of the command – this instructs erdpy to dump the output of the operation in the specified file. The output contains the address of the newly deployed contract and the hash of the deployment transaction.
counter.json { "emitted_tx": { "tx": { "nonce": 0, "value": "0", "receiver": "erd1qqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqq6gq4hu", "sender": "erd1...", "gasPrice": 1000000000, "gasLimit": 5000000, "data": "MDA2MTczNmQwMTAwMDA...MDA=", "chainID": "T", "version": 1, "signature": "a0ba..." }, "hash": "...", "data": "...", "address": "erd1qqqqqqqqqqqqqpgqp93y6..." } }
Feel free to inspect these values in the Explorer.
Interact with the deployed contract
Let’s extract the contract address counter.json
before proceeding to actual contract execution.
export CONTRACT_ADDRESS=$(python3 -c "import json; data = json.load(open('counter.json')); print(data['emitted_tx']['address'])")
Now that we have the contract address saved in a shell variable, we can call the increment
function of the contract as follows:
erdpy --verbose contract call $CONTRACT_ADDRESS --pem="alice.pem" --gas-limit=2000000 --function="increment" --proxy="https://testnet-gateway.elrond.com" --recall-nonce --send
Execute the command above a few times, with some pause in between. Then feel free to experiment with calling the decrement
function.
Then, in order to query the value of the counter – that is, to execute the get
pure function of the contract – run the following:
erdpy contract query $CONTRACT_ADDRESS --function="get" --proxy="https://testnet-gateway.elrond.com"
The output should look like this:
[{'base64': 'AQ==', 'hex': '01', 'number': 1}]
Interaction script
The previous steps can be summed up in a simple script as follows:
#!/bin/bash # Deployment erdpy --verbose contract deploy --project=mycounter --pem="alice.pem" --gas-limit=5000000 --proxy="https://testnet-gateway.elrond.com" --outfile="counter.json" --recall-nonce --send export CONTRACT_ADDRESS=$(python3 -c "import json; data = json.load(open('address.json')); print(data['emitted_tx']['contract'])") # Interaction erdpy --verbose contract call $CONTRACT_ADDRESS --pem="alice.pem" --gas-limit=2000000 --function="increment" --proxy="https://testnet-gateway.elrond.com" --recall-nonce --send sleep 10 erdpy --verbose contract call $CONTRACT_ADDRESS --pem="alice.pem" --gas-limit=2000000 --function="increment" --proxy="https://testnet-gateway.elrond.com" --recall-nonce --send sleep 10 erdpy --verbose contract call $CONTRACT_ADDRESS --pem="alice.pem" --gas-limit=2000000 --function="decrement" --proxy="https://testnet-gateway.elrond.com" --recall-nonce --send sleep 10 # Querying erdpy contract query $CONTRACT_ADDRESS --function="get" --proxy="https://testnet-gateway.elrond.com"
Setup a Local Testnet
Prerequisites: erdpy
In order to install erdpy, follow the instructions at install erdpy.
ERDPY VERSION
Make sure your erdpy version is 1.3.2
or higher.
Prerequisites: Node and Proxy
Run the following command, which will fetch the prerequisites (elrond-go
, elrond-proxy-go
, golang
and testwallets
) into ~/elrondsdk
:
$ erdpy testnet prerequisites
Testnet Configuration
Let’s configure the following network parameters in erdpy, so that subsequent command invocations (of erdpy) will not require you explicitly provide the --proxy
and --chainID
arguments:
$ erdpy config set chainID local-testnet $ erdpy config set proxy http://localhost:7950
Then, in a folder of your choice add a file names testnet.toml
with the content below.
$ mkdir MySandbox && cd MySandbox $ touch testnet.toml
testnet.toml [networking] port_proxy = 7950
TIP
erdpy allows you to customize the configuration of the local mini-testnet in much greater detail, but for the sake of simplicity, in the example above we’ve only set the TCP port of the Elrond Proxy.
Then, configure and build the local testnet as follows:
$ cd MySandbox $ erdpy testnet config
Upon running this command, a new folder called testnet
will be added in the current directory. This folder contains the Node & Proxy binaries, their configurations, plus the development wallets.
WARNING
The development wallets (Alice, Bob, Carol, …, Mike) are publicly known – they should only be used for development and testing purpose.
The development wallets are minted at genesis and their keys (both PEM files and Wallet JSON files) can be found in the folder testnet/wallets/users
.
Starting the Testnet
erdpy testnet start
This will start the Seednode, the Validators, the Observers and the Proxy.
IMPORTANT
Note that the Proxy starts with a delay of about 30 seconds.
Sending transactions
Let’s send a simple transaction using erdpy:
Simple Transfer erdpy tx new --recall-nonce --data="Hello, World" --gas-limit=70000 \ --receiver=erd1spyavw0956vq68xj8y4tenjpq2wd5a9p2c6j8gsz7ztyrnpxrruqzu66jx \ --pem=~/elrondsdk/testwallets/latest/users/alice.pem \ --send
You should see the prepared transaction and the transaction hash in the stdout
(or in the --outfile
of your choice). Using the transaction hash, you can query the status of the transaction against the Proxy or against erdpy itself:
$ curl http://localhost:7950/transaction/1dcfb2227e32483f0a5148b98341af319e9bd2824a76f605421482b36a1418f7 $ erdpy tx get --hash=1dcfb2227e32483f0a5148b98341af319e9bd2824a76f605421482b36a1418f7
Deploying and interacting with Smart Contracts
Let’s deploy a Smart Contract using erdpy. We’ll use the simple Counter as an example.
If you need guidance on how to build the Counter sample contract, please follow the Counter SmartContract Tutorial.
Deploy Contract erdpy --verbose contract deploy --bytecode=./counter.wasm \ --recall-nonce --gas-limit=5000000 \ --pem=~/elrondsdk/testwallets/latest/users/alice.pem \ --outfile=myCounter.json \ --send
Upon deployment, you can check the status of the transaction and the existence of the Smart Contract:
$ curl http://localhost:7950/transaction/0db61bab8e78779ae009300988c6be0949086d93e2b7adfddd5e6375a4b6eeb7 | jq $ curl http://localhost:7950/address/erd1qqqqqqqqqqqqqpgqj5zftf3ef3gqm3gklcetpmxwg43rh8z2d8ss2e49aq | jq
If everything is fine (transaction status is executed
and the code
property of the address is set), you can interact with or perform queries against the deployed contract:
Call Contract erdpy --verbose contract call erd1qqqqqqqqqqqqqpgqj5zftf3ef3gqm3gklcetpmxwg43rh8z2d8ss2e49aq \ --recall-nonce --gas-limit=1000000 --function=increment \ --pem=~/elrondsdk/testwallets/latest/users/alice.pem --outfile=myCall.json \ --send
Query Contract erdpy --verbose contract query erd1qqqqqqqqqqqqqpgqj5zftf3ef3gqm3gklcetpmxwg43rh8z2d8ss2e49aq --function=get
Simulating transactions
At times, you can simulate transactions instead of broadcasting them, by replacing the flag --send
with the flag --simulate
. For example:
Simulate: Call Contract all-nonce --gas-limit=1000000 --function=increment \ --pem=~/elrondsdk/testwallets/latest/users/alice.pem --outfile=myCall.json \ --simulate
Simulate: Simple Transfer erdpy tx new --recall-nonce --data="Hello, World" --gas-limit=70000 \ --receiver=erd1spyavw0956vq68xj8y4tenjpq2wd5a9p2c6j8gsz7ztyrnpxrruqzu66jx \ --pem=~/elrondsdk/testwallets/latest/users/alice.pem \ --simulate