Advanced
How to set up a local development environment for Celo using Windows and deploy a smart contract to Celo testnet, mainnet, or a local network using Truffle.
Welcome to this tutorial on how to set up a local development environment for Celo using Windows and deploy a smart contract to Celo testnet, mainnet, or a local network using Truffle. In this tutorial, we will be using the Windows Subsystem for Linux (WSL) to install a Linux distribution, set up the Linux environment, and develop with WSL. We will also be using the Truffle suite to create and deploy our smart contract. By following the steps outlined in this tutorial, you will be able to set up a local development environment and deploy a smart contract to the Celo network of your choice.
Getting set up with Windows
Open PowerShell as an administrator and run
Enable-WindowsOptionalFeature -Online -FeatureName Microsoft-Windows-Subsystem-Linux
Restart your computer when prompted.
Next, install a Linux distribution from the Microsoft Store. When developing this guide, we chose Ubuntu.
Set up your Linux distro by setting a username and password then update and upgrade the packages by running the following command in the terminal.
sudo apt update && sudo apt upgrade
You can view the source documentation for setting up the Linux distro here and the Microsoft documentation for setting up the Windows Subsystem for Linux here.
Set up the Linux Environment
Now that you have Linux installed, let’s install nvm and yarn. Nvm (node version manager) makes it easy to install and manage different versions of Node.js. The following instructions are from the celo-monoreop setup documentation for Linux.
Run the following commands in the Linux terminal.
# Installing Nvm curl -o- https://raw.githubusercontent.com/nvm-sh/nvm/v0.39.1/install.sh | bash source ~/.bashrc # Setting up the desired Nvm version nvm install x nvm alias default x
Running $ node -v in the terminal should print a node version if it is installed correctly.
Yarn is a package manager similar to npm. The celo-monorepo uses yarn to build and manage packages. Install yarn with the following command.
# Installing Yarn - https://yarnpkg.com/en/docs/install#debian-stable curl -sS https://dl.yarnpkg.com/debian/pubkey.gpg | sudo apt-key add - echo "deb https://dl.yarnpkg.com/debian/ stable main" | sudo tee /etc/apt/sources.list.d/yarn.list sudo apt-get update && sudo apt-get install yarn
Test that yarn is installed by running $ yarn –version.
Developing with WSL
You can now start working on your projects in your Linux environment. Install the WSL VS Code extension for a seamless integration between VS Code and WSL.
Be aware that networking will be different depending on which version of WSL you are using. The details of managing network interfaces goes beyond the scope of this guide, but you can learn more here.
Install truffle using this link – Truffle
Project Setup
Setup Project Folder
Open your terminal window, create a project directory, and navigate into that directory.
mkdir myDapp && cd myDap
Install hdwallet-provider
From your root truffle project directory, install truffle/hdwallet-provider. This allows you to sign transactions for addresses derived from a mnemonic. You’ll use this to connect to Celo in your truffle configuration file.
npm install @truffle/hdwallet-provider --save
Initialize Truffle
Initializing truffle creates the scaffolding for your truffle project.
truffle init
Open Project
Open your project in Visual Studio code or your preferred IDE.
code .
Write Project Code
Create Smart Contract
Create a file named HelloCelo.sol in the contracts directory and populate it with the Solidity code below.
// SPDX-License-Identifier: MIT pragma solidity ^0.8.3; contract HelloWorld { string public greet = "Hello Celo!"; }
Migrations File
Create a file named 2_deploy_contracts.js in the ./migrations/ folder and populate it with the code below.
var HelloCelo = artifacts.require("HelloCelo"); module.exports = function (deployer) { deployer.deploy(HelloCelo); };
Mnemonic .env
If you don’t yet have a mnemonic or are unsure how to create one, see Set up a Development Wallet for more details. When you’re ready, create a .env file in your root directory and populate it with your development wallet mnemonic (example below).
MNEMONIC="turtle cash neutral drift brisk young swallow raw payment drill mail wear penalty vibrant entire adjust near chapter mistake size angry planet slam demand"
This mnemonic is used by HDWalletProvider in the truffle.config file to verify the account supplying funds during contract deployment. (See lines 21 & 69.)
Create .gitignore file
It is important to hide your mnemonic and other important files while developing Celo applications. When using Git or GitHub, you can populate a .gitignore file with the code below to ensure you don’t accidentally publish these files.
Create a .gitignore file in your root directory and populate it with the code below.
# dependencies /node_modules # Mac users .DS_Store #hidden files .env
Configure Deployment Settings
The default truffle.config.js file contains connections required to deploy to the Ethereum networks, imports HDWalletProvider, and connects to the mnemonic in your .env file. To deploy a Celo network, you need to update this configuration file to point toward the different Celo networks and add a few details specific to Celo best practices.
You can see an example repo here.
Update the truffle-config.js file
Open truffle-config.js in a text editor and replace its contents with this Celo configuration code. This code is similar to Truffle settings with a few configuration updates needed to deploy to a Celo network.
Connect to a Development Network
Using Celo Ganache CLI creates test accounts at the localhost on port 7545. The private network setup connects to your localhost on this port and gives you access to your accounts on ganache-cli.
local: { host: "127.0.0.1", port: 7545, network_id: "*" }
Connect to Testnet using Forno
Using Forno allows you to connect to the Celo test blockchain without running a local node. The testnet configuration uses Forno to connect you to the Celo Testnet (Alfajores) using HDWalletProvider and the mnemonic stored in your .env file.
testnet: { provider: function() { return new HDWalletProvider(process.env.MNEMONIC, "https://alfajores-forno.celo-testnet.org") }, network_id: 44787, gas: 20000000 }
Connect to Mainnet using Forno
Using Forno also allows you to connect to the Celo main blockchain without running a local node. The mainnet configuration uses Forno to connect you to the Celo Mainnet using HDWalletProvider and the mnemonic stored in your .env file.
mainnet: { provider: function() { return new HDWalletProvider(process.env.MNEMONIC, "https://forno.celo.org") }, network_id: 42220, gas: 4000000 }
Deploying Smart Contract
Compile and Migrate
Compile Contract
Compile the Solidity code into Ethereum bytecode prior to deploying the contract. The following truffle command will compile any new or updated Solidity (.sol) contracts found in ./contracts.
truffle compile
Migrate Contract
Migrations are JavaScript files that help you deploy contracts to the Ethereum network. To run your migrations, run the following:
truffle migrate
Deploy Contract
Deploy to your chosen Celo network running one of the following commands.
truffle deploy --network alfajores
truffle deploy --network celo
truffle deploy --network local
Deploy with reset —
truffle deploy --network NETWORK --reset
truffle migrate --network NETWORK --reset
View Contract Deployment
Copy your contract address from the terminal and navigate to the block explorer to search for your deployed contract. Switch between networks to find your contract using the dropdown by the search bar.
View Deployed Contract
Navigate to BlockScout and select the network of your deployed contract.
-
Paste your contract address from the terminal window and search for it in BlockExplorer.
Verify Smart Contract using Celo Explorer
Verifying a smart contract allows developers to review your code from within the Celo Block Explorer.
- Navigate to the Code tab at the Explorer page for your contract’s address
- Click Verify & Publish to enter the smart contract verification page
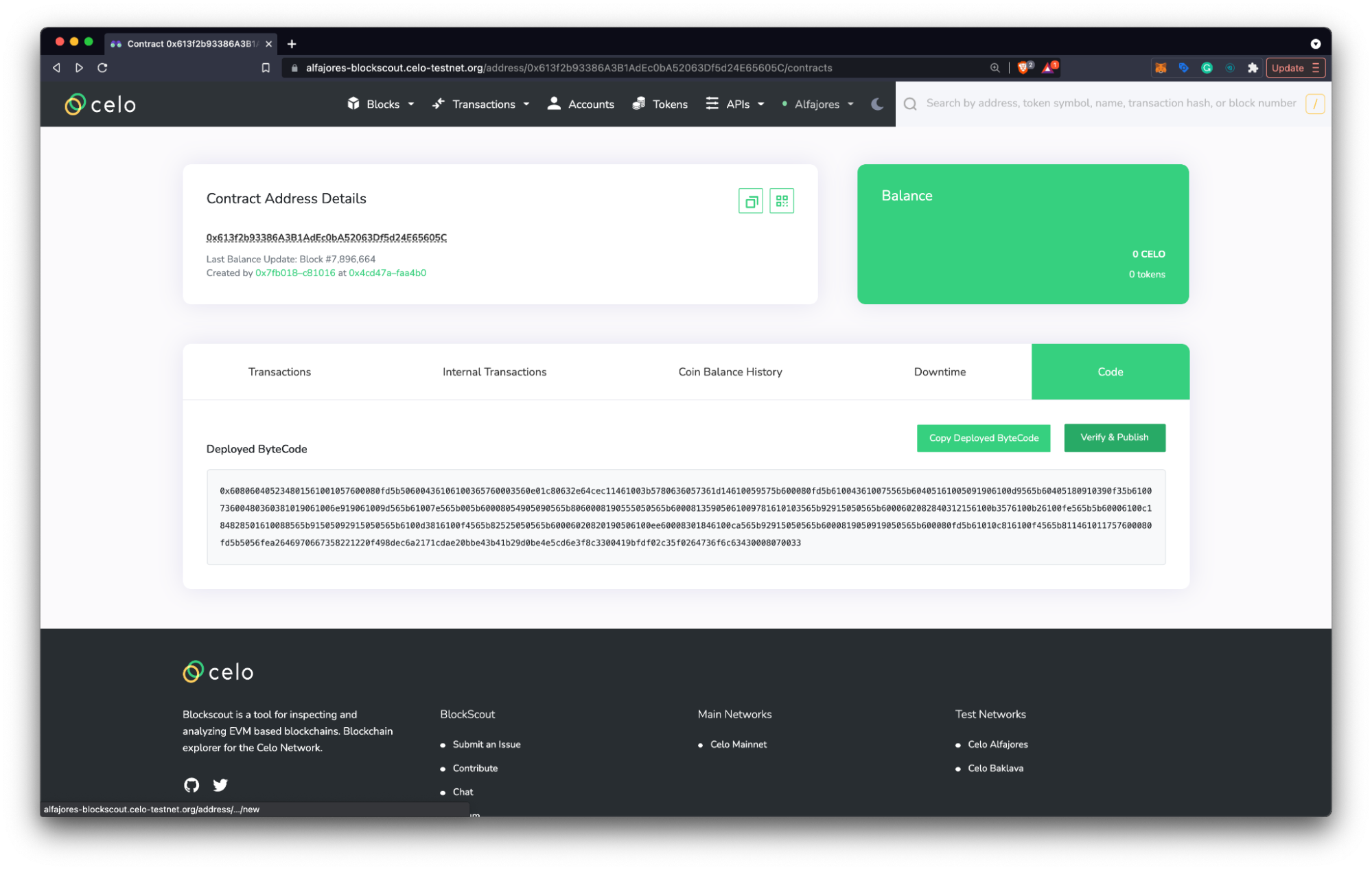
- Upload your smart contract (example: HelloCelo.sol) and it’s .json file (example: HelloCelo.json) found in build > contracts folder.
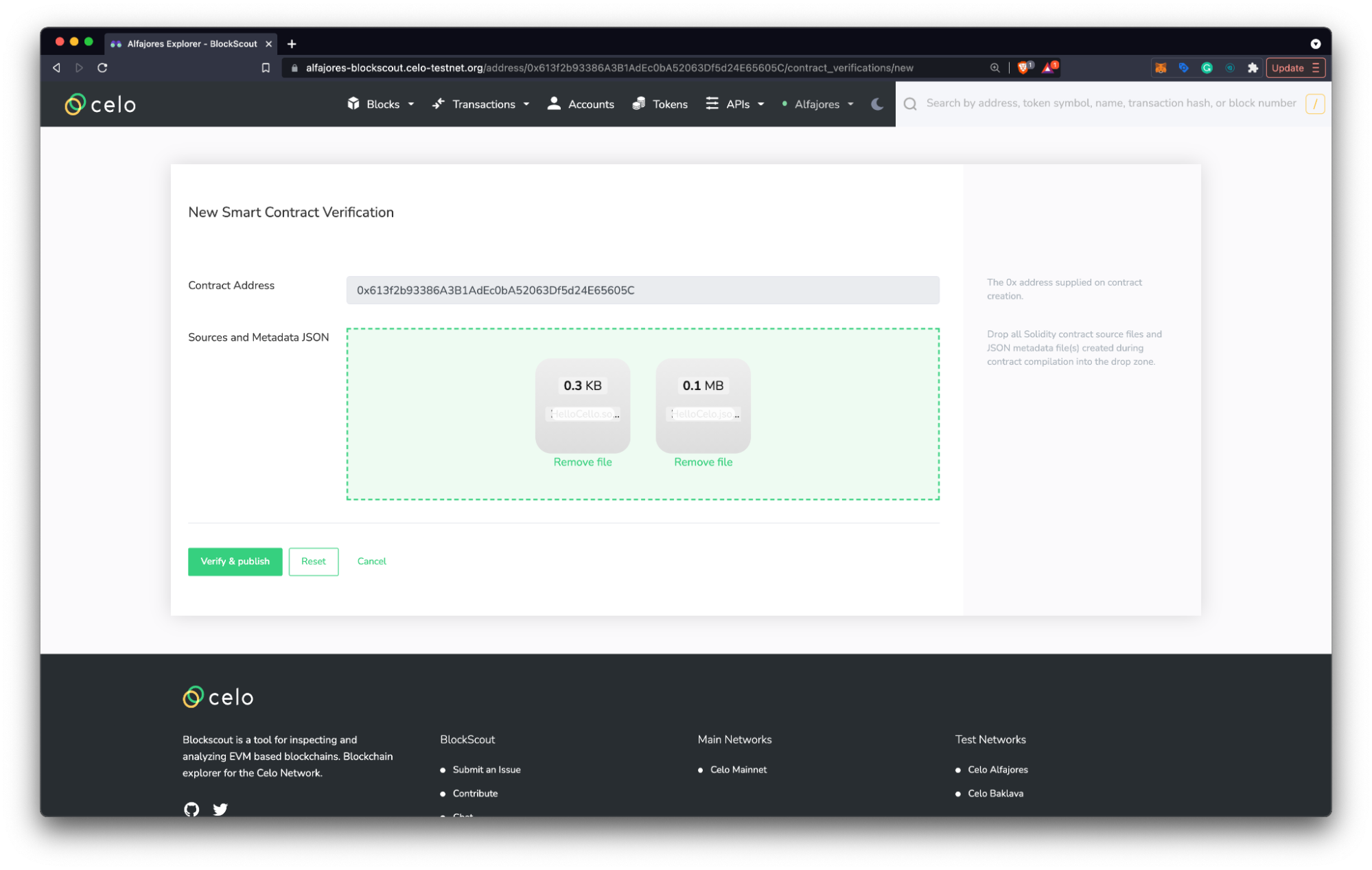
- Click Verify & Publish
- Navigate to the Contract Address Details Page in the block explore to, use the Code, Read Contract, and Write Contract panels to view and interact with your deployed smart contract.